Introduction
Python provides various features. Let's look at a few built-in functions:
- print() – for data output;
- abs() – to return the absolute value of a number;
- int() – to convert another data type to an integer;
- len() – returns the length of a sequence or collection.
Modules must be used, due to limited functionality. We connect external modules and thus get more functions.
Modules in Python have a “.py” extension at the end of the file. You can refer to functions, classes, or variables in other modules using the Python command line.
When we import the test module, the interpreter looks for the built-in module. If it doesn't find it, it looks for the test.py file in the directory it gets from the sys.path variable.
This tutorial will cover checking, installing, importing and changing long names of modules with functions.
Preparing for import
Need to use our instruction, to prepare in advance before starting work. You need to update the package index and update the software packages that are required.
Important! Run as a user with sudo access. If you work on a production server, so as not to harm your data in the server.
Checking for Installing Modules
After installing the Python update, we get access to a number of modules. The modules are installed with Python and the standard library for minified tasks.
To test Python on the command line, type:
python3
The command interpreter will open and after “>>>” you will be able to perform various functions.
To check, we activate the virtual environment with:
source /testing/testingv/bin/activate
python3
The name will be prefixed with (testingv) as shown and enter the Python shell as shown in Screen 1.

We import two modules "math" and "matplotlib". Math - is a built-in module for mathematical calculations and the command should be executed without error. Matplotlib is a library for working with 2D graphics, we can see the results in Screen 2.

Exit the shell and use pip3 (the command interpreter for installing libraries) to install matplotlib.
exit() – exit from the command interpreter;
pip3 install matplotlib
The result is shown in Screen 3.
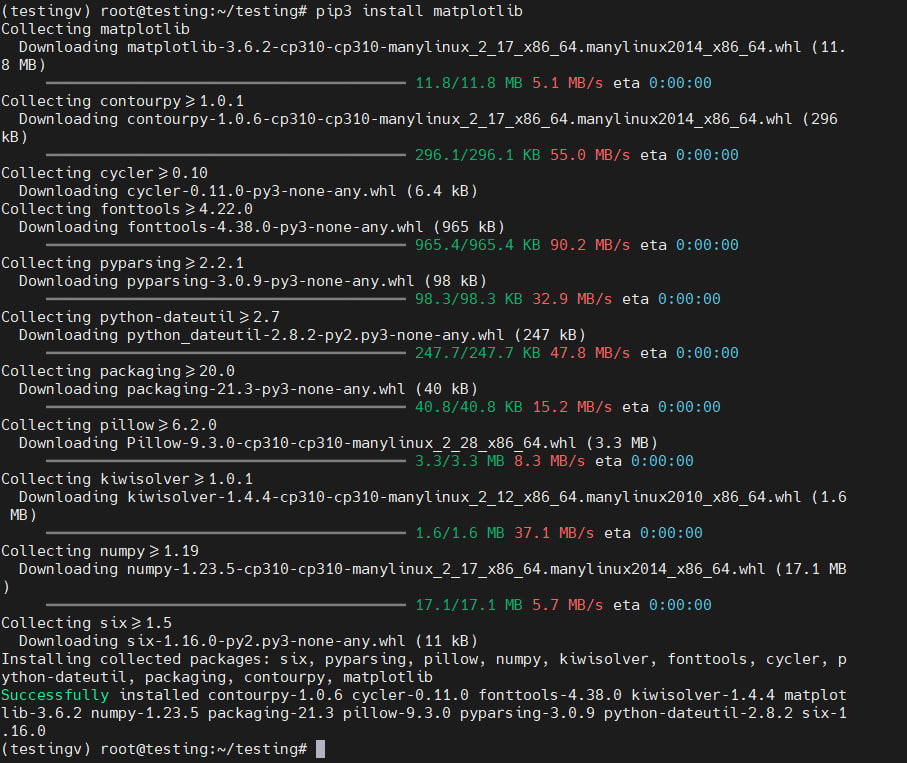
Let's try to re-import the modules again and get a successful result, as shown in Screen 4.
import math
import matplotlib

We consider the first step completed. We were able to install the module using pip3 and did the import successfully.
Importing modules
Let's exit the shell and create the testing_random.py file. The line with importing modules is inserted at the very top of the code or under the shebang. The shebang is #!/usr/bin/env python3 - execute the file as a Python program, using the environment to get the path to the interpreter file. Import the random library to generate random numbers.
#!/usr/bin/env python3
import random
By importing modules, access to its functions is opened. Before executing the function, to begin with, the name of the module is indicated, a period is put and the name of the function is indicated. Consider an example of generating random numbers 7 times from 5 to 15 using the randint function, as shown in the following lines of code.
import random
print("Output:")
for i in range(7):
print(random.randint(5, 15))
Let's run the file with "python3 testing_random.py". At the output, we get 7 columns with different generated values. For example, in our case, this is the following data:
Output:
6
14
14
12
12
12
12
At the same time, please note that the data should not be lower than 5 and not higher than 15. In case of using other modules, you should import other modules at the beginning of the code.
import random
import math
Let's edit the code, when receiving the result, we will indicate 7 columns of the generated data from 5 to 15, and add the output number Pi.
import random
import math
print("Output:")
for i in range(7):
print(random.randint(5,15))
print(math.pi)
When executing this code, we get the following data:
Output:
9
13
12
15
7
14
13
3.141592653589793
Also with the help of import it is possible to import several modules at once.
Using from ... import
The from statement is useful because we don't have to specify the module name before using the function. This process simplifies the speed of writing code. At the beginning, we specify which function we import from the module and write the code. Consider a simplified version of our previous code and the result is shown in Screen 5:
from math import pi
from random import randint
print("Output")
for i in range(7):
print(randint(5,15))
print(pi)

It's safe to say that importing a few functions from a module at the beginning of the code saves a lot more time than naming the module and then naming the function in the code.
Changing the name of the module and functions
In Python, it is perfectly possible to replace a module name with a shorter name by using "as" at the beginning of the code. This case must be used if the module name already exists in the code. You can also replace the module with a long name, which we use more often. An example is shown in the following line of code:
import math as m
print(m.pi)
print(m.e)
The result is shown in Screen 6.

Output of numbers of constants Pi and Exponent.
You can also use "as" to shorten the name matplotlib.pyplot, for example to pyplt. You can see in official guide on using the matplotlib module.
Conclusions
The instructions covered options such as:
- Module import;
- Using a function using the name of the added module;
- Importing a function from a module;
- Using a function without a module name;
- Changing the name of the module;