Programming languages are used to solve all kinds of problems related to massive data types, such as lists, strings, tuples, dictionaries. And many others that require a modern language to handle a large incoming stream of data. In Python for working with such types there are loops, which are divided into a loop with a counter for and without a certain number of steps while python loop. The second one will be discussed today!
What is While in Python?
While - is a cyclic construct that is executed as long as the condition specified for it is true. Inside the construct there are instructions to execute the loop, as well as keywords to stop the construct itself or skip iteration. Let's consider its syntax below:
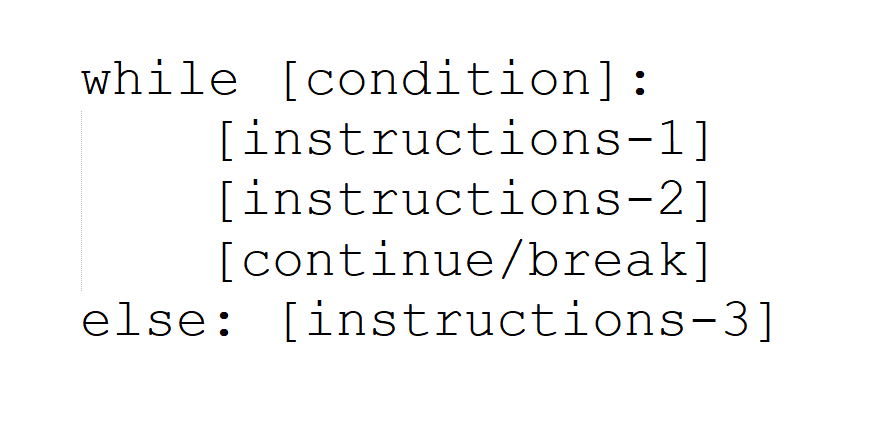
First, the while keyword is defined, with a condition that is checked for truth and if it is True, a number of instructions are executed. Note that everything that is indented belongs to the construct and will be executed according to the loop rule, except for continue/break, but we will talk about it below. From theory to practice, and if before it was not clear how the loop machine works, the example will make it clear. For example, we need to output numbers from 0 to 5 for this we will write the construction below:
n=0
while n <= 5:
print (n)
n+=1
print ( 'END')
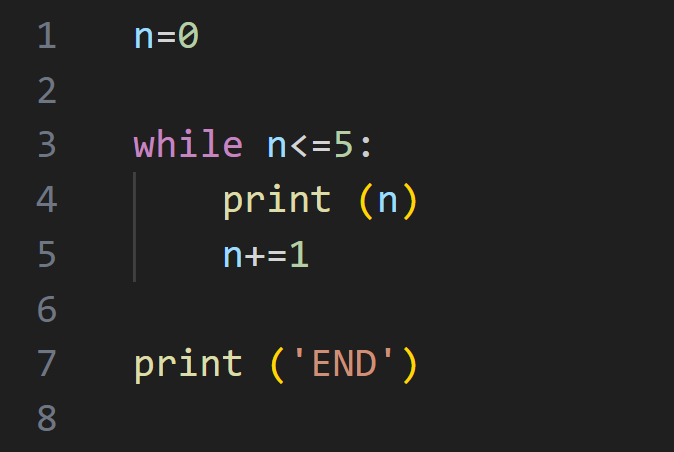
Note that not all parts of the cyclic construct from the syntax have been used, because the presented ones are enough to solve this problem. Let's explain how the loop works: first, n becomes zero, then control goes to the while loop, where the construct ‘As long as n is less than or equal to 5, do it!’ is literally executed. The Python language is fairly human-readable, so it's easy enough to understand logically.
Back to the loop, since zero is greater than five, the condition will execute and go to print our variable n with a value of zero. It will also add one to it, and then the control will go to while again and check the condition. This round from check to check is called iteration. As soon as the variable n is equal to 6, the condition will not be fulfilled and the control will switch to print (‘END’). The output will look like this:
0
1
2
3
4
5
END
As you can see this solution is much longer than the numerical rights designation, however, it is just as functional and fulfils the tasks. All steps in the tutorial can be performed on powerful cloud servers. Serverspace provides isolated VPS / VDS servers for common and virtualize usage.
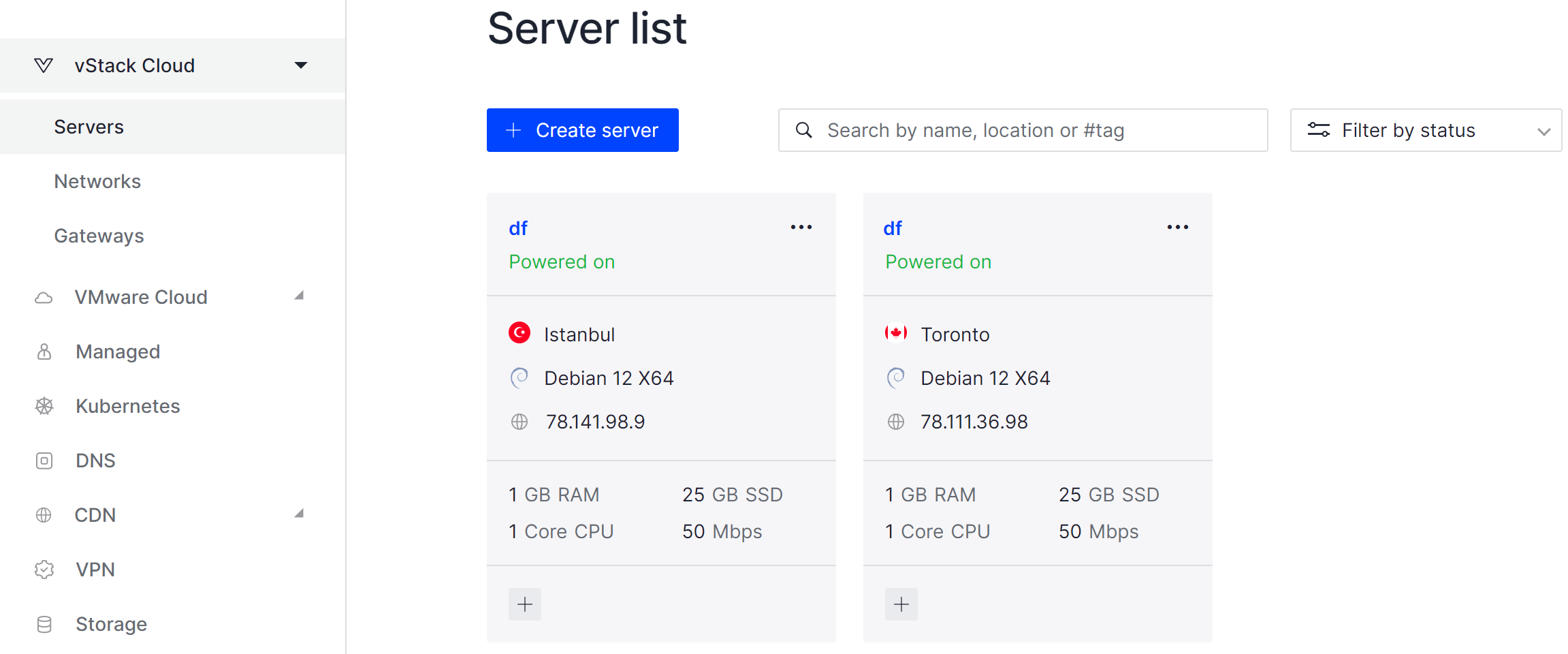
It will take some time to deploy server capacity. After that you can connect in any of the convenient ways.
Back to loops, since loops are just a construct, they can take any Python instructions inside them, which increases the possibilities of working with them. However, it is important to remember that you can define an algorithm whose condition will always be true and it will not stop on its own. For example:
n=True
while n:
print (n)
print ('END')
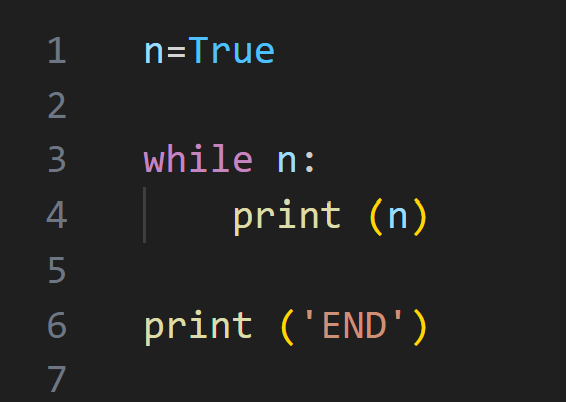
A condition error in this algorithm causes the python infinite loop to loop around and print True. After all, the python loop variable n stores it and does not change in the process, which means that print (‘END’) will never occur, because control will not be passed from the loop. Therefore, it is advisable to avoid algorithms with a non-changing condition.
Block else in a while loop
In some cases it may be necessary to display additional notification to users, change values, and reset variables after the loop. Else is just for this purpose and will be executed when the condition is False or not fulfilled. Let's notify users that the loop search has ended:
user_language = input('Your languages:')
ex_language =['en', 'fr', 'jp', 'kz']
while user_language in ex_language:
print (f'Language found in the list: {user_language}')
user_language = 0
else: print ('End of search!')
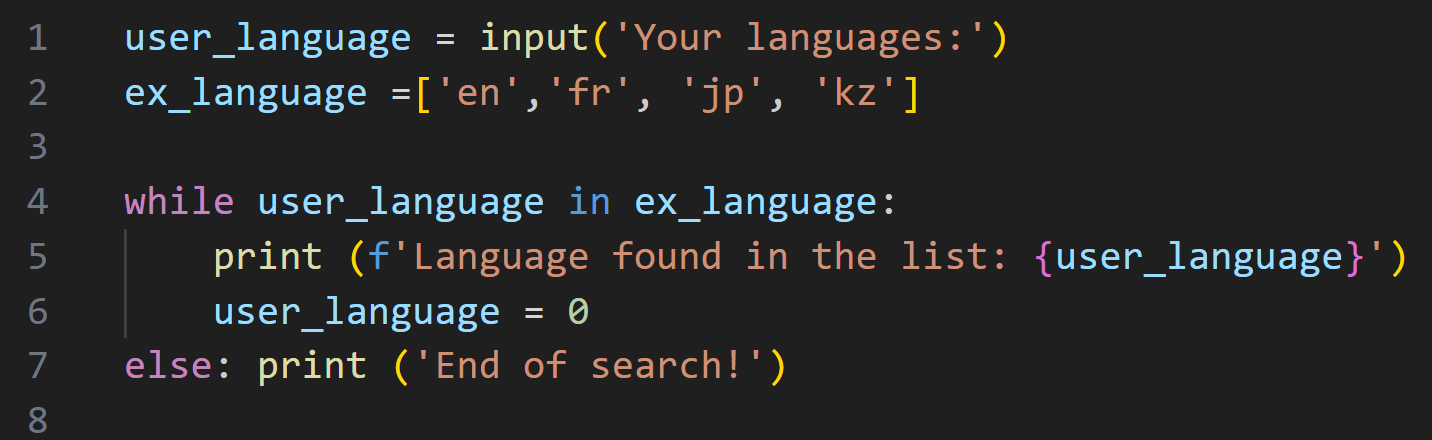
If the language entered by the user is in the list of available languages, it will be found and displayed. And after the condition is invalid it will pass control to the else block and write the line ‘End of search!’.
And how can I force the loop to stop after the received value?
Break and continue blocks in Python
For this purpose, there are blocks that allow you to end the loop execution - break, skip the current iteration - continue. They are used in the same way as regular keywords, but instructions are not executed after them, which is important to take into account when writing code. Let's look at an example:
user_language = input('Your languages:')
ex_language =['en','fr', 'jp', 'kz']
while user_language in ex_language:
if user_language == 'jp':
user_language='0'
continue
print (f'Language found in the list: {user_language}')
break
else: print ('The language could not be found!')
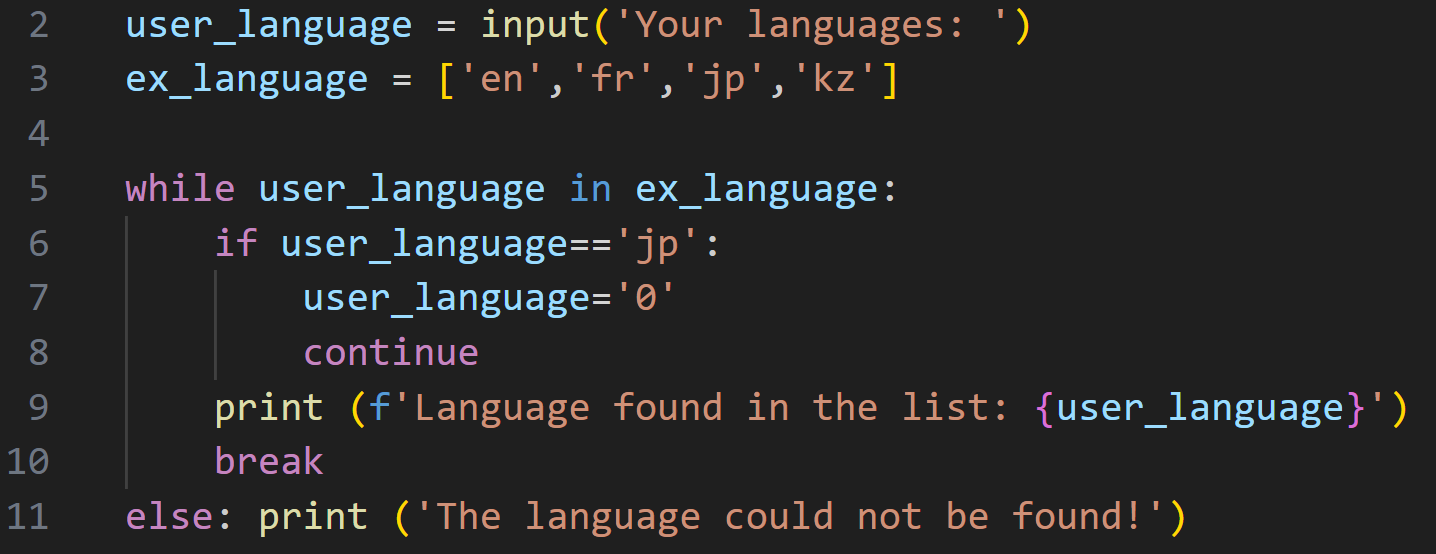
If the user enters the jp language, passing the while control it will soon pass to the conditional if construct, where the logical expression is also checked. In the case of the current value of jp, the condition is correct and the actions of the conditional construct will be executed, namely zeroing user_language and continue, which will end the current iteration and proceed to the new one. This means that the condition will be checked again and displayed on the screen:
Your languages: jp
Language not found in the list!
However, if you enter another value from the list of languages, for example en, the loop will work with the output:
Your languages: en
Found language in the list: en
Since after passing control to break, the loop is terminated without checking again and else is not executed because it is part of the loop. Understanding and properly using the while loop allows you to efficiently solve problems related to repetitive processes and data processing in Python, making your code more flexible and powerful.